Render and combine PDF files into one
Render PDF files from HTML templates in Rails can be done using WickedPDF.
First you need to use ActionView
to render HTML to a string:
def render_to_string(data)
action_view = ActionView::Base.new
action_view.view_paths = ActionController::Base.view_paths
action_view.class_eval do
include ApplicationHelper
include PDFHelper
# or other helpers
end
action_view.render template: 'pdf/template.html',
layout: 'layout/pdf.html',
locals: { data: data }
end
Then you can render pdf with the desired settings like this:
# first pdf file with some view settings and values from data1...
pdf1 = WickedPdf.new.pdf_from_string(
render_to_string(data1), {
pdf: 'report1',
page_size: 'Letter',
orientation: 'Portrait'
})
# ...and second pdf file with some view settings and values from data2
pdf2 = WickedPdf.new.pdf_from_string(
render_to_string(data2), {
pdf: 'report2',
page_size: 'Letter',
orientation: 'Landscape'
})
And now you can combine it with CombinePDF gem, that provide you parse
method to get PDF content and to_pdf
method to render the result back to PDF.
combiner = CombinePDF.new
combiner << CombinePDF.parse(pdf1)
combiner << CombinePDF.parse(pdf2)
combiner.to_pdf
Discover More Reads
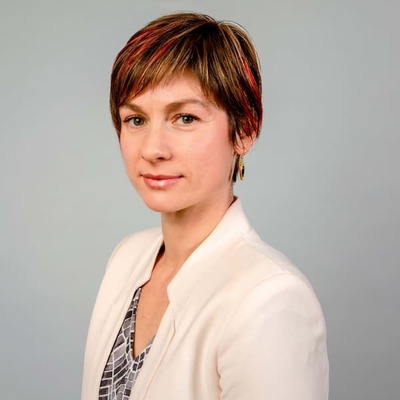
Categories: